This post offers a variety of zoom functions to help you analyze charts better. For example, you can zoom in on the cursor-selected bar, or you can step forward and backward from signal to signal or over custom price conditions. These functions are made possible by the ZoomToRange OLE function, which lets you zoom in on a specific date range.
To exercise and test the functions below, copy and Insert the code to an Indicator pane, open the Param window, and click on various options to see how the functions work. For demo purposes, three zoom events are provided: Buy, Sell, and Mondays. In your own application, you would assign other conditions to the event variable, such as, for example, gaps, price patterns, etc. Stepping from Event to Event would allow you to visually analyze the chart surrounding events. The Param window looks like this:
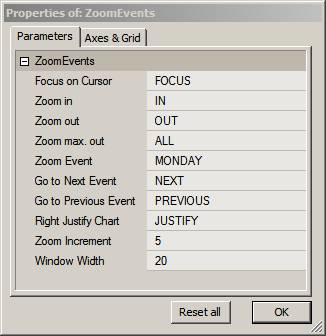
Zoom Increment is the number of bars added to the display with each zoom in/out action. WindowWidth is the number of bars displayed when jumping from event to event.
The program uses a FocusBar to which zoom dimensions are referenced. Yellow text is attached to the FocusBar on the chart to identify it. You can add other information to this PlotText(). For example, if you were analyzing buy, sell, short, and cover signals, you could add trade prices and indicator values, if you so desired. The Green histogram at the bottom of the chart indicates where the selected events occur. See an example chart below showing buy signals. The green histogram bar occurs just before the green buy arrow on the chart.
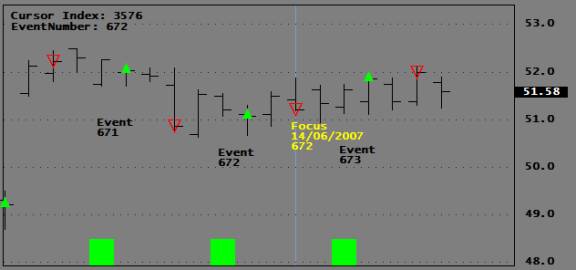
Descriptions for the function below are presented ahead of the functions themselves so that you can copy all the code in one step.
ZoomToIndex( FirstBarIndex, LastBarIndex)
Calling the ZoomToIndex() will zoom your chart to display a window ranging from the first argument (FirstBarIndex) to the second argument (LastBarIndex). While you cannot zoom in closer than ten bars using the Zoom In/Out buttons on your AB Toolbar, this function lets you zoom all the way down to a two-bar window. Since most Indicators use periods expressed in bars and not in dates, the function uses the BarIndex to set the window width.
ZoomToCursor( ZoomWidth )
This function lets you zoom in to the cursor position and display the number of bars assigned to ZoomWidth. The values of important variables are displayed in the chart Title for educational purposes.
ZoomOut( ZoomWidth )
This function zooms out by increasing the number of bars displayed by ZoomWidth bars.
ZoomIn( ZoomWidth )
This function zooms in by reducing the number of bars displayed by ZoomWidth bars.
ZoomToNext( Event, ZoomWidth )
This function lets you jump to the next occurrence of a TRUE value in the Event Array. It also focuses on the event with a window of ZoomWidth bars. The most important application for this function would probably be to analyze trades by stepping from signal to signal and to visually inspect custom events or price patterns.
ZoomToPrev( Event, ZoomWidth )
This function is similar to the one above, but it jumps (retreats) to the previous event.
MarkFocus()
This function places the Yellow text at the FocusBar. You may want to customize the text displayed.
ZoomAllOut()
This function zooms out to display all bars in the database.
RightJustifyChart
When trading Real-Time, your signals are generated by prices from the Last Bar whether this bar is visible or not. If, during intense trading, you miss the fact that your Chart is not fully right justified, you may see and hear orders going out while no actions show on your Chart. While such confusion usually doesn’t last long, it could delay discretionary trades and even trick you into thinking there may be a coding problem, causing you to initiate debugging code. This function right-justifies your chart, and if it is triggered at the start of your session, it will prevent you from working with a shifted chart.
procedure ZoomToIndex( FirstBarIndex, LastBarIndex)
{
StaticVarSet("FirstBarIndex",FirstBarIndex);
StaticVarSet("LastBarIndex",LastBarIndex);
DT = DateTime();
BI = BarIndex();
LastDateTime = LastValue(ValueWhen( LastBarIndex == BI, DT ));
FirstDateTime = LastValue(ValueWhen( FirstBarIndex == BI, DT ));
LastDateTimestr = DateTimeToStr( LastDateTime );
FirstDateTimestr = DateTimeToStr( FirstDateTime );
AB = CreateObject("Broker.Application");
AW = AB.ActiveWindow;
AW.ZoomToRange( FirstDateTimestr, LastDateTimestr );
}
procedure ZoomToCursor( ZoomWidth )
{
local ZoomWidth;
BI = BarIndex();
SBI = SelectedValue(BI);
LBI = LastValue(BI);
CursorIndex= Nz(StaticVarGet("CursorIndex"));
FirstBarIndex = Max(0,SBI - ZoomWidth);
LastBarIndex = Min( LBI, SBI + ZoomWidth );
ZoomToIndex( FirstBarIndex, LastBarIndex);
}
procedure ZoomOut( ZoomWidth )
{
local ZoomWidth;
BI = BarIndex();
LBI = LastValue(BI);
LastVisiblebar = Status("LastVisibleBar");
FirstVisibleBar= Status("FirstVisibleBar");
FirstBarIndex = Max( 0, FirstVisibleBar - ZoomWidth);
LastBarIndex = Min( LBI, LastVisibleBar + ZoomWidth );
ZoomToIndex( FirstBarIndex, LastBarIndex);
}
procedure ZoomIn( ZoomWidth )
{
local ZoomWidth;
_TRACE("##");
BI = BarIndex();
LBI = LastValue(BI);
LastVisiblebar = Status("LastVisibleBar");
FirstVisibleBar= Status("FirstVisibleBar");
FirstBarIndex = Max( 0, FirstVisibleBar + ZoomWidth);
LastBarIndex = Min( LBI, LastVisibleBar - ZoomWidth );
ZoomToIndex( FirstBarIndex, LastBarIndex);
}
procedure ZoomToNext( Event, ZoomWidth )
{
local Event, Zoomwidth;
EventNum = Cum(Event);
BI = BarIndex();
LBI = LastValue(BI);
DT=DateTime();
NextEventNum = Min(Nz(StaticVarGet("EventNumber"))+1, LastValue(EventNum));
NextEventIndex = LastValue(ValueWhen(EventNum == NextEventNum, BI));
StaticVarSet("EventNumber",Max(1, NextEventNum));
FirstBarIndex = Max( 0, NextEventIndex - ZoomWidth );
LastBarIndex = Min( BarCount-1, NextEventIndex + ZoomWidth);
ZoomToIndex( FirstBarIndex, LastBarIndex);
StaticVarSet("FocusIndex",NextEventIndex);
}
procedure ZoomToPrev( Event, ZoomWidth )
{
local Event, Zoomwidth;
EventNum = Cum(Event);
BI = BarIndex();
LBI = LastValue(BI);
LastEventNum = LastValue(EventNum);
NextEventNum = Max(1,Nz(StaticVarGet("EventNumber"))-1);
NextEventIndex = LastValue(ValueWhen(EventNum == NextEventNum, BI,1));
StaticVarSet("EventNumber",NextEventNum);
FirstBarIndex = Max( 0, NextEventIndex - ZoomWidth );
LastBarIndex = Min( BarCount-1, NextEventIndex + ZoomWidth);
ZoomToIndex( FirstBarIndex, LastBarIndex);
StaticVarSet("FocusIndex",NextEventIndex);
}
procedure MarkFocus()
{
global EventNum;
DT=DateTime();
BI = BarIndex();
LBI = LastValue(BI);
FirstBarIndex = Nz(StaticVarGet("FirstBarIndex"));
FocusIndex = Min(LBI,Nz(StaticVarGet("FocusIndex"))+1);
FocusDateStr = NumToStr(DT[FocusIndex],formatDateTime);
ENStr = NumToStr(EventNum[FocusIndex],1.0,False);
PlotText("\n\nFocus\n"+FocusDateStr+"\n"+ENStr,FocusIndex,C[FocusIndex],colorYellow);
}
procedure ZoomAllOut()
{
BI = BarIndex();
LBI = LastValue(BI);
FirstBarIndex = 0;
LastBarIndex = LBI;
ZoomToIndex( FirstBarIndex, LastBarIndex);
}
procedure RightJustifyChart( ChartWidth )
{
DT = DateTime();
BI = BarIndex();
FirstDateTime = LastValue( Nz(Ref( DT,-Max(2, ChartWidth) ) ) );
FirstDateTimestr = DateTimeToStr( FirstDateTime );
LastDateTimestr = DateTimeToStr( LastValue(DT) );
AB = CreateObject("Broker.Application");
AW = AB.ActiveWindow;
AW.ZoomToRange( FirstDateTimestr, LastDateTimestr );
}
SetBarsRequired(1000000,1000000);
GraphXSpace = 20;
_SECTION_BEGIN("ZOOM");
FocusTrigger= ParamTrigger("Focus on Cursor","FOCUS");// zoom out by ZoomIncrement
ZoomInTrigger= ParamTrigger("Zoom in","IN");// zoom in by ZoomIncrement
ZoomOutTrigger= ParamTrigger("Zoom out","OUT");// zoom in by ZoomIncrement
ZoomAllTrigger = ParamTrigger("Zoom max. out","ALL");// zoom out to all bars
ZoomPrevTrigger= ParamTrigger("Go to Next Event","NEXT");// Jump to next event
ZoomNextTrigger= ParamTrigger("Go to Previous Event","PREVIOUS");// Jump to previous event
RJTrigger= ParamTrigger("Right Justify Chart","JUSTIFY");
ZoomIncrement= Param("Zoom Increment",10,1,1000,2);
ZoomWidth= Param("Window Width",20,2,1000,1);
_SECTION_END();
_SECTION_BEGIN("TEST SIGNALS");
ZoomEvent= ParamList("Zoom Event","MONDAY|RSI-BUY|RSI-SELL|TARGET",1);// Test events
ProfitTarget= Param("Profit Target",0.1,0,2,0.01);// Profit target
_SECTION_END();
PrevZoomWidth= Nz(StaticVarGet("ZoomWidth"));
ZoomChange= PrevZoomWidth != ZoomWidth;
StaticVarSet("ZoomWidth",ZoomWidth);
ZoomAction = FocusTrigger OR ZoomOutTrigger OR ZoomAllTrigger OR ZoomNextTrigger OR ZoomPrevTrigger OR ZoomChange OR ZoomInTrigger OR RJTrigger;
if( ZoomEvent == "MONDAY" ) Event = DayOfWeek() == 1;
else if( ZoomEvent == "RSI-BUY" )
{
Event = Cross(30,RSI());// Buy rule to investigate
PlotShapes(IIf(Event,shapeSmallUpTriangle, shapeNone),5,0,C,0);
Sell = 0;
}
else if( ZoomEvent == "RSI-SELL" )
{
Event = Cross(RSI(),70);// sell rule to investigate
PlotShapes(IIf(Event,shapeHollowDownTriangle, shapeNone),4,0,C,0);
Buy = 0;
}
else if( Zoomevent == "TARGET" )
{
Target = Ref(C,1) - C;// Profit target to analyze
Event = Target > ProfitTarget;
PlotShapes(IIf(Event,shapeSmallUpTriangle, shapeNone),5,0,C,0);
}
else Event = 0;
Plot(C,"",1,128);
EventNum = Cum(Event);
FVBI = Status("FirstVisibleBarIndex");
LVBI = Status("LastVisibleBarIndex");
BI = BarIndex();
SBI = SelectedValue(BI);
LBI = LastValue(BI);
FirstVisibleBar = Status( "FirstVisibleBar" );
Lastvisiblebar = Status("LastVisibleBar");
for( b = Firstvisiblebar; b < Lastvisiblebar AND b < BarCount; b++)
{
if( Event[b] ) PlotText("\n\n\n\nEvent\n"+NumToStr(EventNum[b],1.0,False),b,L[b],1);
}
if( GetCursorMouseButtons() == 1 )
{
StaticVarSet("CursorIndex",SBI);
EventNum = Cum(Event);
StaticVarSet("EventNumber",SelectedValue(EventNum));
StaticVarSet("FocusIndex",SBI-1);
}
CursorIndex= Nz(StaticVarGet("CursorIndex"));
if( ZoomAction )
{
CurrentRange= LastVisiblebar-FirstVisibleBar;
VisibleCenter= int((LastVisibleBar + FirstVisibleBar)/2);
PrevEventIndex = LastValue(ValueWhen(Event,BI,1));
NextEventIndex = LastValue(ValueWhen(Event,BI,-1));
FirstBarIndex = Nz(StaticVarGet("FirstBarIndex"));
LastBarIndex = Nz(StaticVarGet("LastBarIndex"));
if( FocusTrigger) ZoomToCursor( ZoomWidth );
else if( ZoomInTrigger ) ZoomIn( ZoomWidth );
else if( ZoomOutTrigger ) ZoomOut( ZoomWidth );
else if( ZoomNextTrigger ) ZoomToPrev( Event, ZoomWidth );
else if( ZoomPrevTrigger ) ZoomToNext( Event, ZoomWidth );
else if( ZoomAllTrigger ) ZoomAllOut();
else if( RJTrigger ) RightJustifyChart( ZoomWidth );
}
MArkFocus();
Plot(Event,"",5,styleArea|styleOwnScale|styleNoLabel,0,10);
CursorIndex = StaticVarGet("CursorIndex");
EventNumber= StaticVarGet("EventNumber");
Title = "\n"+
"Cursor Index: "+NumToStr(CursorIndex,1.0,False)+"\n"+
"EventNumber: "+NumToStr(EventNumber,1.0,False)+"\n";
Edited by Al Venosa